MySQLに配列を格納したい
Windows10 + MySQL8.0 + Python3.8.5
MySQLに配列を入れたかったがMySQLには配列型がないらしい。
配列を何かしらの形に変換して入れざるを得ない。
JSON型を扱えるようなので配列をJSON形式に変換することにする。
1. 実装
1.1. 事前準備
前回の記事を参考に最低限必要なコードを書く。
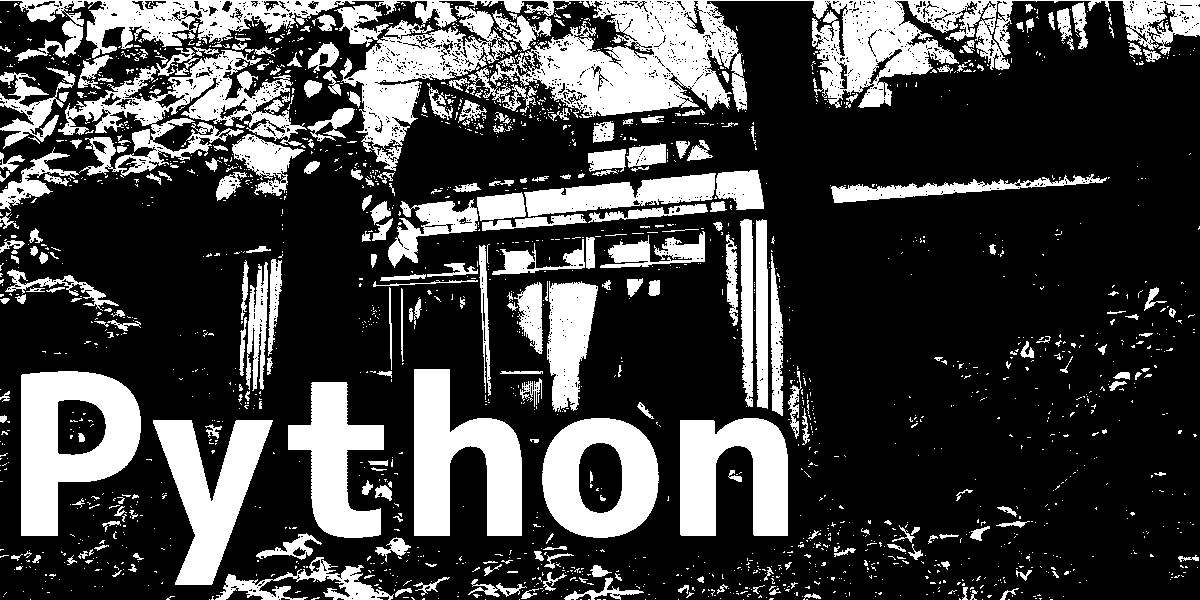
1 | import mysql.connector as mydb |
さらに今回はJSON形式のファイルを扱うのでjson
ライブラリを追加する。
1 | import json |
1.2. テーブルを作る
json_test
という名前のテーブルを作る。json_test
にはint型で自動的に番号が振られるid
と、json型のdata
が含まれる。auto_increment
を使うために必要らしいのでprimary key(id)
としている。
同じ名前のテーブルがすでにある時に何もしないためにif not exists
としている。
1 | cur.execute("create table if not exists json_test(id integer auto_increment, data json, primary key (id))") |
1.3. データを保存する
json.dumps()
でリストをJSON形式に変換する。
これを文字列としてSQL文の中に入れる。
ここで、%s
で展開するだけだと文字列として扱われないので前後に\'
としてシングルクォーテーションを付ける。
1 | data = [1.23, 4.56, 7.89] |
ここでndarray
をjson.dumps()
に入れるとObject of type ndarray is not JSON serializable
とエラーが出る。
単純にjson.dumps(array.tolist())
というようにtolist()
でリストに変換したら良い。
1.4. データを参照する
単純にselect * from json_test
とcur.fetchall()
で取得したデータはレコードが1行ごとタプルに入ったリストになっている。
今回のテーブルは2列目にJSONデータが入っているため、任意の行でrow[1]
を取り出す。
取り出したJSONデータをjson.loads()
で読み込むことで元のリストが取得できる。
1 | # 全てのデータを取得・表示 |